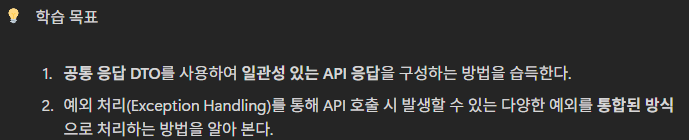
ApiUtil 클래스 작성
- ApiUtil 클래스는 모든 API 응답을 동일한 형태로 구성하기 위한 공통 응답 DTO입니다.
- 이를 통해 성공 응답과 에러 응답을 일관된 구조로 제공할 수 있습니다.
package com.tenco.blog_v3.common.utils;
import lombok.Data;
@Data
public class ApiUtil<T> {
private Integer status; // 협의 - 1, 성공 -1 실패
private String msg;
private T body;
public ApiUtil(T body) {
this.status = 200;
this.msg = "성공";
this.body = body;
}
public ApiUtil(Integer status, String msg) {
this.status = status;
this.msg = msg;
this.body = null;
}
}
- T: 제네릭 타입으로, 응답 본문(body)에 담을 데이터의 타입을 유연하게 처리합니다.
- 성공 응답: status는 200, msg는 "성공"으로 설정되고, 본문에는 실제 데이터가 담깁니다.
- 에러 응답: status와 msg는 에러에 맞는 코드와 메시지로 설정되며, 본문은 비워둡니다.
우리가 사용할 에러 코드 확인
- 400: 잘못된 요청
- 401: 인증되지 않은 사용자
- 403: 권한 없음
- 404: 자원 없음
- 500: 서버 내부 오류
package com.tenco.blog_v3.common;
import com.tenco.blog_v3.common.errors.*;
import com.tenco.blog_v3.common.utils.ApiUtil;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
// 데이터 반환
@RestControllerAdvice
public class GlobalExceptionHandler {
// 400 에러 처리 - return - json
@ExceptionHandler(Exception400.class)
public ResponseEntity<?> handleException400(Exception400 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(400, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception401.class)
public ResponseEntity<?> handleException401(Exception401 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(401, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.UNAUTHORIZED);
}
@ExceptionHandler(Exception403.class)
public ResponseEntity<?> handleException403(Exception403 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(403, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.FORBIDDEN);
}
@ExceptionHandler(Exception404.class)
public ResponseEntity<?> handleException404(Exception404 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(404, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception500.class)
public ResponseEntity<?> handleException500(Exception500 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(500, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
- @RestControllerAdvice: 모든 컨트롤러에서 발생한 예외를 감지하고, 해당 예외에 대한 응답을 처리하는 역할을 합니다.
- @ExceptionHandler: 특정 예외 클래스가 발생할 때 실행되는 메서드를 지정합니다.
- 예외가 발생하면, ApiUtil을 사용해 클라이언트에게 에러 메시지와 HTTP 상태 코드를 전달합니다.
'Spring boot > 개념 공부' 카테고리의 다른 글
JWT란 뭘까? (0) | 2024.11.08 |
---|---|
RestAPI 컨트롤러 요청과 응답 (1) | 2024.11.08 |
RestAPI 주소 변경 및 인터셉터 수정 (0) | 2024.11.08 |
뷰 연결 컨트롤러 정리 (0) | 2024.11.08 |
RestAPI 주소 설계 규칙 (0) | 2024.11.08 |
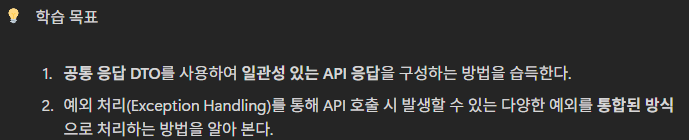
ApiUtil 클래스 작성
- ApiUtil 클래스는 모든 API 응답을 동일한 형태로 구성하기 위한 공통 응답 DTO입니다.
- 이를 통해 성공 응답과 에러 응답을 일관된 구조로 제공할 수 있습니다.
package com.tenco.blog_v3.common.utils;
import lombok.Data;
@Data
public class ApiUtil<T> {
private Integer status; // 협의 - 1, 성공 -1 실패
private String msg;
private T body;
public ApiUtil(T body) {
this.status = 200;
this.msg = "성공";
this.body = body;
}
public ApiUtil(Integer status, String msg) {
this.status = status;
this.msg = msg;
this.body = null;
}
}
- T: 제네릭 타입으로, 응답 본문(body)에 담을 데이터의 타입을 유연하게 처리합니다.
- 성공 응답: status는 200, msg는 "성공"으로 설정되고, 본문에는 실제 데이터가 담깁니다.
- 에러 응답: status와 msg는 에러에 맞는 코드와 메시지로 설정되며, 본문은 비워둡니다.
우리가 사용할 에러 코드 확인
- 400: 잘못된 요청
- 401: 인증되지 않은 사용자
- 403: 권한 없음
- 404: 자원 없음
- 500: 서버 내부 오류
package com.tenco.blog_v3.common;
import com.tenco.blog_v3.common.errors.*;
import com.tenco.blog_v3.common.utils.ApiUtil;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
// 데이터 반환
@RestControllerAdvice
public class GlobalExceptionHandler {
// 400 에러 처리 - return - json
@ExceptionHandler(Exception400.class)
public ResponseEntity<?> handleException400(Exception400 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(400, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception401.class)
public ResponseEntity<?> handleException401(Exception401 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(401, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.UNAUTHORIZED);
}
@ExceptionHandler(Exception403.class)
public ResponseEntity<?> handleException403(Exception403 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(403, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.FORBIDDEN);
}
@ExceptionHandler(Exception404.class)
public ResponseEntity<?> handleException404(Exception404 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(404, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception500.class)
public ResponseEntity<?> handleException500(Exception500 e) {
ApiUtil<?> apiUtil = new ApiUtil<>(500, e.getMessage());
return new ResponseEntity<>(apiUtil, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
- @RestControllerAdvice: 모든 컨트롤러에서 발생한 예외를 감지하고, 해당 예외에 대한 응답을 처리하는 역할을 합니다.
- @ExceptionHandler: 특정 예외 클래스가 발생할 때 실행되는 메서드를 지정합니다.
- 예외가 발생하면, ApiUtil을 사용해 클라이언트에게 에러 메시지와 HTTP 상태 코드를 전달합니다.
'Spring boot > 개념 공부' 카테고리의 다른 글
JWT란 뭘까? (0) | 2024.11.08 |
---|---|
RestAPI 컨트롤러 요청과 응답 (1) | 2024.11.08 |
RestAPI 주소 변경 및 인터셉터 수정 (0) | 2024.11.08 |
뷰 연결 컨트롤러 정리 (0) | 2024.11.08 |
RestAPI 주소 설계 규칙 (0) | 2024.11.08 |